Guides overview
Learn the basics of our developer platform.
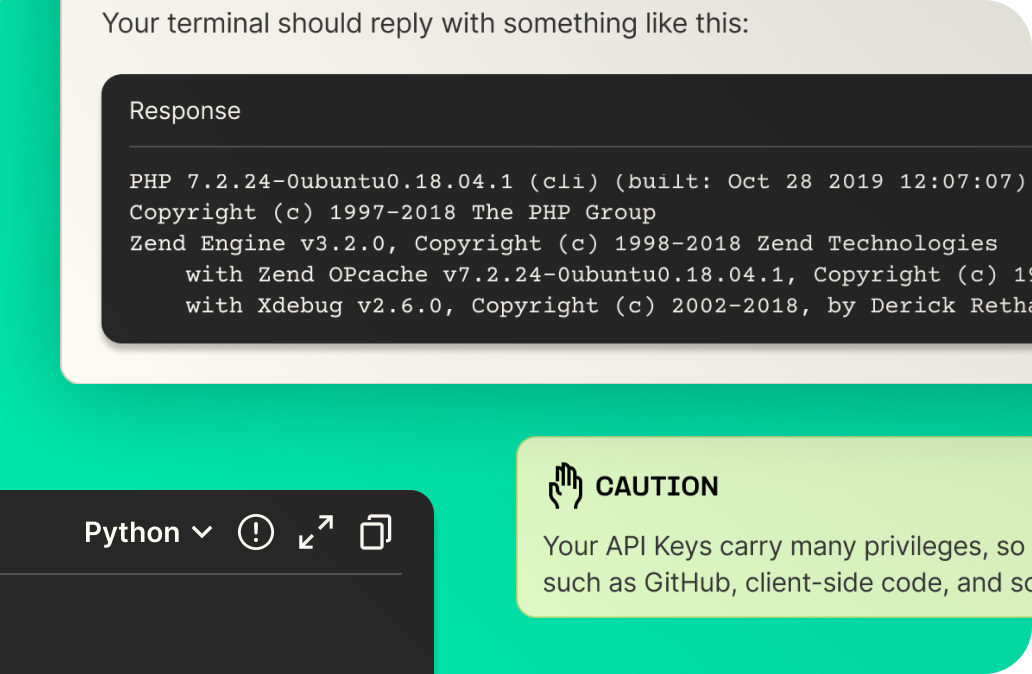
To start building with Telnyx, you’ll need to sign up for an account on our Mission Control Portal, as well as perform a few tasks below, including: buying a phone number, creating a SIP Connection, creating an Outbound Voice Profile, and obtaining your API Keys.
To quickly jump to authentication, setups, etc., use the jump links in the menu at right.
Sign up for a Telnyx account
To start building, you’ll need to create an account to access our Mission Control Portal, where you can buy numbers, set up and manage SIP Connections, and more. Head to telnyx.com/sign-up to create your free Telnyx account.
Buy a phone number
You can search for, buy, and provision new numbers, or port existing numbers all within the Numbers section of the Telnyx Portal.
Simply click on "Numbers", then either "Search & Buy Numbers" or "Port Numbers" and follow the prompts.
Create a SIP connection
Select SIP Connections on the left-hand navigation menu, and click "+Add SIP Connection." Then, set up your Connection authentication mode and any expert settings you'd like. Now, go to your number and add the Connection you just created.
PRO TIPYou can also do this programmatically via our RESTful API. Check out our documentation for SIP Connections.
Create an Outbound Voice profile
Select Outbound Voice Profiles on the left-hand navigation menu, click "+Add New Profile" and set up your profile name. Add the SIP Connection you just created above, the traffic type, the service plan, and your desired billing method.
PRO TIPYou can also do this programmatically via our RESTful API. Check out our documentation for Outbound Voice Profiles.
Obtain your API keys
When you’re ready to start developing with our APIs, you’ll need your API Keys. Telnyx authenticates your API requests using your account’s API Key. If you do not include your key when making an API request or use a key that’s incorrect or outdated, Telnyx returns an error.
Your API Keys are available in the Auth section of the Portal. Once you create an API Key you must save it for future use. You will only see your API Key once, on creation.
Note: Your API Keys carry many privileges, so be sure to keep them secure! Do not share your secret API Keys in publicly accessible areas such as GitHub, client-side code, and so forth.
We include YOUR_API_KEY
in our code examples. Replace this text with your own API Key.
All API requests must be made over HTTPS. Calls made over plain HTTP will fail. API requests without authentication will also fail. Below is an example of a cURL authenticated request:
curl -X GET \
--header "Authorization: Bearer YOUR_API_KEY" \
--globoff "https://api.telnyx.com/v2/messaging_profiles?page[size]=10"
Note: If you cannot see your API keys in the Portal, this means you do not have access to them. Only Telnyx account owners can access API Keys.
That’s it! You're all set to start integrating Telnyx with your applications. Next steps: Check out our configuration guides here or learn how to set up your development environment in the section below to start building your own application.
Developer setup
After completing the steps above, you’re ready to interact with our APIs directly. Please explore our full reference of APIs and check out our Postman collection. If you would like to utilize a server SDK to simplify API interactions, proceed forward with the language of your choice:
Python | PHP | Node | Java | .NET | Ruby
Install Telnyx Python SDK
You'll need to ensure that you have Python installed on your computer. If Python isn’t installed, follow the official installation instructions for your operating system to install it. You can check this by running the following:
python --version
NoteAfter pasting the above content, Kindly check and remove any new line added
You’ll need to have pip installed. If pip isn’t installed, follow the official pip installation instructions for your operating system to install it. You can check this by running:
pip --version
Install the Telnyx Python SDK by running the following:
pip install telnyx
Check out the source on GitHub.
Install Telnyx PHP SDK
Make sure that you have at least PHP version 5.3 installed on your computer, as this is the minimum version of PHP that the SDK requires; we recommended you to always use the latest version of PHP. If PHP isn’t installed, follow the official installation instructions for your operating system to install it. You can check this by running the following:
php --version
NoteAfter pasting the above content, Kindly check and remove any new line added
Your terminal should reply with something like this:
PHP 7.2.24-0ubuntu0.18.04.1 (cli) (built: Oct 28 2019 12:07:07) ( NTS )
Copyright (c) 1997-2018 The PHP Group
Zend Engine v3.2.0, Copyright (c) 1998-2018 Zend Technologies
with Zend OPcache v7.2.24-0ubuntu0.18.04.1, Copyright (c) 1999-2018, by Zend Technologies
with Xdebug v2.6.0, Copyright (c) 2002-2018, by Derick Rethans
You’ll also need to have composer installed. Composer is PHP's package manager. While it's not a part of the PHP installation itself, it’s a de-facto standard that virtually every modern PHP library uses. If composer isn’t installed, follow the official composer installation instructions for your operating system to install it. You can check this by running:
composer --version
SDKs and other libraries are always installed for each project as a dependency and stored in a project-specific directory called vendor
that Composer manages.
There are two ways to add a package with Composer:
Using the Require Command
Create a new project directory or open an existing project directory in your terminal. Then, type the following command:
composer require telnyx/telnyx-php
This command installs the latest version of the SDK and automatically creates or updates the files composer.json
and composer.lock
.
Using composer.json
Create a new project directory or open an existing project directory. In this directory, create a file called composer.json
; if you already have this file, you can add the SDK to it; if you only have the SDK in it, the file should look like this:
{
"require": {
"telnyx/telnyx-php": "^0.0.1"
}
}
Keep in mind that you have to specify the version of the SDK in the file. You can see the available SDK versions on Packagist and learn more about different ways to specify versions, for example with ranges, in the Composer documentation.
After saving the file, open a terminal in the directory into which you've stored it and type this command:
composer install
Check out the source on GitHub.
Install Telnyx Node SDK
You'll need to ensure that you have Node.js installed on your computer. If Node isn’t installed, follow the official installation instructions for your operating system to install it. You can check this by running the following:
node --version
NoteAfter pasting the above content, Kindly check and remove any new line added
You’ll need to have npm installed (npm is installed with Node.js). You can check this by running:
npm --version
Install the Telnyx Node SDK by running the following:
npm install telnyx
Check out the source on GitHub.
Install Telnyx Java SDK
You'll need to ensure that you have at least Java JDK 1.8 installed on your computer. If Java isn’t installed, follow the installation instructions for your operating system. You can check this by running the following:
java -version
NoteAfter pasting the above content, Kindly check and remove any new line added
Check out the source on GitHub, or see Package on Maven Central.
Install the Telnyx SDK using Maven or Gradle.
The latest version can be found on our releases page.
Maven
Add the snip to your pom.xml file.
<dependency>
<groupId>com.telnyx.sdk</groupId>
<artifactId>telnyx</artifactId>
<version>{version}</version>
</dependency>
Gradle
Run the command
compile "com.telnyx.sdk:telnyx:{version}"
Send message example
To get started:
-
Sign up for a Telnyx Account
-
Get your API Key
-
Complete the messaging Learn and Build to:
- Get your first phone number
- Create your messaging profile
-
Fill in the variables:
YOUR_TELNYX_NUMBER
,YOUR_TELNYX_API_KEY
,YOUR_MOBILE_NUMBER
in the code below
Demo below instantiates the Telnyx client
import com.telnyx.sdk.ApiClient;
import com.telnyx.sdk.ApiException;
import com.telnyx.sdk.Configuration;
import com.telnyx.sdk.auth.*;
import com.telnyx.sdk.model.*;
import com.telnyx.sdk.api.MessagesApi;
public class Example {
private static final String YOUR_TELNYX_NUMBER = "+19842550944";
private static final String YOUR_MOBILE_NUMBER = "+19198675309";
private static final String YOUR_TELNYX_API_KEY = "YOU_API_KEY";
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://api.telnyx.com/v2");
// Configure HTTP bearer authorization: bearerAuth
HttpBearerAuth bearerAuth = (HttpBearerAuth) defaultClient.getAuthentication("bearerAuth");
bearerAuth.setBearerToken(YOUR_TELNYX_API_KEY);
MessagesApi apiInstance = new MessagesApi(defaultClient);
// CreateMessageRequest | Message payload
CreateMessageRequest createMessageRequest = new CreateMessageRequest()
.from(YOUR_TELNYX_NUMBER)
.to(YOUR_MOBILE_NUMBER)
.text("Hello From Telnyx");
try {
MessageResponse result = apiInstance.createMessage(createMessageRequest);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling MessagesApi#createMessage");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
Webhook verification:
This example is dependent on the Bouncy Castle library. Visit our Webhook Verification Demo for more information.
[...]
@RestController
public class WebhookController {
private static final Dotenv dotenv = Dotenv.load();
private final String TELNYX_PUBLIC_KEY = dotenv.get("TELNYX_PUBLIC_KEY");
@PostMapping("/messaging/inbound")
public ResponseEntity<Void> webhook(@RequestBody String payload, @RequestHeader HttpHeaders headers) {
Security.addProvider(new BouncyCastleProvider());
String signature, timestamp;
try {
signature = headers.get("telnyx-signature-ed25519").get(0);
timestamp = headers.get("telnyx-timestamp").get(0);
}
catch (Exception e) {
return new ResponseEntity(HttpStatus.UNAUTHORIZED);
}
String signedPayload = timestamp + "|" + payload;
byte[] signedPayloadBytes = signedPayload.getBytes(StandardCharsets.UTF_8);
Ed25519PublicKeyParameters ed25519PublicKeyParameters = new Ed25519PublicKeyParameters(Base64.getDecoder().decode(TELNYX_PUBLIC_KEY), 0);
Signer verifier = new Ed25519Signer();
verifier.init(false, ed25519PublicKeyParameters);
verifier.update(signedPayloadBytes, 0, signedPayloadBytes.length);
boolean isVerified = verifier.verifySignature(Base64.getDecoder().decode(signature));
if(isVerified) {
return new ResponseEntity(HttpStatus.OK);
} else {
return new ResponseEntity(HttpStatus.UNAUTHORIZED);
}
}
}
Install Telnyx .NET SDK
You'll need to ensure that you have DotNet installed on your computer. If DotNet (core or framework) isn’t installed, follow the official installation instructions for your operating system to install it. You can check this by running the following:
Telnyx targets the .NET Standard 2.0. You should be running a supported verison of dotnet/Xamarin/Mono/Framework to leverage the SDK.
dotnet --version
NoteAfter pasting the above content, Kindly check and remove any new line added
Install the Telnyx.net SDK by running the following:
dotnet add package Telnyx.net
Check out the source on GitHub, or see Package on NuGet
Send message async example
The C# SDK supports Task based API calls.
By updating the Main method to support async calls, we can build a quick example.
Demo below instantiates the Telnyx client and sends a "Hello, World!" message to the outbound phone number then prints the message ID.
Be sure to update the From
& To
numbers to your Telnyx number and destination phone number respectively.
using System;
using Telnyx;
using System.Threading.Tasks;
namespace demo_dotnet_telnyx
{
class Program
{
private static string TELNYX_API_KEY = System.Environment.GetEnvironmentVariable("TELNYX_API_KEY");
static async Task Main(string[] args)
{
Console.WriteLine("Hello World!");
TelnyxConfiguration.SetApiKey(TELNYX_API_KEY);
MessagingSenderIdService service = new MessagingSenderIdService();
NewMessagingSenderId options = new NewMessagingSenderId
{
From = "+19198675309", // alphanumeric sender id
To = "+19198675310",
Text = "Hello, World!"
};
MessagingSenderId messageResponse = await service.CreateAsync(options);
Console.WriteLine(messageResponse.Id);
}
}
}
Install Telnyx Ruby SDK
You'll need to ensure that you have Ruby installed on your computer. If Ruby isn’t installed, follow the official installation instructions for your operating system to install it. You can check this by running the following:
ruby --version
NoteAfter pasting the above content, Kindly check and remove any new line added
You’ll need to have RubyGems installed. If RubyGems isn’t installed, follow the official RubyGems installation instructions for your operating system to install it. You can check this by running:
gem --version
Install the Telnyx Ruby Gem by running the following:
gem install telnyx
Check out the source on GitHub.
Now that you've set up your development environment, you're ready to authenticate your API requests with API Keys.
Authentication
The Telnyx API V2 uses API Keys to authenticate requests. You can view and manage your API Keys in the Auth section of your Mission Control portal.
CAUTIONYour API Keys carry many privileges, so be sure to keep them secure! Do not share your secret API Keys in publicly accessible areas such as GitHub, client-side code, and so forth.
Using API keys
To use your API Key, assign it in your SDK as shown in this quickstart guide section. Using our RESTful API, you can also make requests by passing the API Key in the Authorization
header: Authorization: Bearer YOUR_API_KEY
.
All API requests must be made over HTTPS. Calls made over plain HTTP will fail. API requests without authentication will also fail. Below is an example of a cURL authenticated request:
curl -X GET \
--header "Authorization: Bearer YOUR_API_KEY" \
--globoff "https://api.telnyx.com/v2/messaging_profiles?page[size]=10"
ngrok setup
This guide will walk you through how to get ngrok up and running on your machine. To test it out with Telnyx webhooks, you'll need to sign up. If you'd like to test out your ngrok instance by receiving a webhook associated with an API-enabled phone call, jump to our Receiving Webhooks in Voice API after you complete the steps below.
ngrok is a popular tunneling tool used to expose a locally running application to the internet. You can download it for free with all of the functionality you need here. This is useful for receiving webhooks to your local applications for testing.
For the sake of this tutorial, we'll assume that your local application is running locally on port 5000
. Now you’ll need the ability to send a request to that port from Telnyx. You can easily do this using ngrok when developing your application.
Sign up for ngrok and follow the setup and installation steps to get up and running. The final step in the process is to start an HTTP tunnel to your application. The instructions specify $ ./ngrok http 80
, which will tunnel traffic to port 80
on your machine. As our application is running on port 5000
, you should use that instead: $ ./ngrok http 5000
.
When you run this command, you should see output similar to the following:
ngrok by @inconshreveable
Session Status online
Account Little Bobby Tables (Plan: Free)
Version 2.3.28
Region United States (us)
Web Interface http://127.0.0.1:4040
Forwarding http://ead8b6b4.ngrok.io -> localhost:5000
Forwarding https://ead8b6b4.ngrok.io -> localhost:5000
Connections ttl opn rt1. rt5 p50 p90
0 0 0.00 0.00 0.00 0.00
ngrok forwarding address
The forwarding addresses will be different for you, but they should still point to localhost:5000
. Copy the https forwarding address, as you'll need it to configure your Mission Control Portal.
Messaging with ngrok
For messaging, webhooks set the webhook URL on your messaging profile from the Telnyx Portal Messaging dashboard. Edit your Messaging Profile by clicking the “Basic Options” button [✎]. Select the “Inbound” section and paste the forwarding address from ngrok into the Webhook URL field. Append /webhooks
to the end of the URL to direct the request to the webhook endpoint in your local application.
Resending webhooks
For now, you'll leave “Failover URL” blank, but if you wanted to have Telnyx resend the webhook — if sending to the Webhook URL fails — you can specify an alternate address in this field.
With ngrok set up, you're ready to start testing the Telnyx API to see what it can do with Postman. Follow the steps below to get started.
Postman setup
Postman is an API platform available as a web or desktop application. A great way to understand an API is to make requests and review the responses. Postman does exactly that by providing a UI for testing and experimenting with API calls without the need to write code. We recommend using Postman to get started quickly and get a taste of Telnyx APIs. You can easily accomplish this by utilizing our Postman collections.
Steps to use these collections:
- Configure your local environment
- Import a collection.
- Send a test request and inspect the responses.
Configure environment
An environment is a set of variables you can use in your Postman requests. You can use environments to group related sets of values together.
By the end of this tutorial, you would have imported and configured environment to use with Telnyx collections.
-
Create an API Key following the below steps.
a. Sign up for a free Telnyx account
b. Navigate to API Keys section and create an API Key by clicking
Create API Key
button.NoteYou need to obtain your API key so Telnyx can authenticate your API requests
c. Copy and save this key in a safe place and don't share it with anyone as it is a sensitive value.
-
Sign up at Postman or Download and Install the Postman application.
-
Once signed in to the Postman, select an existing workspace or create a new workspace for your Telnyx collections. If you are new to postman, learn more about creating a workspace here
-
Once you are in your desired workspace, click Import in the top left corner, select Link from the options and paste this link in the Enter a URL text box:
https://tlyx.co/telnyx-postman-environment
Then click continue and it should show basic details of the environment you are about to import. Click Import and you should see a success confirmation at the bottom right
-
Go to Environments tab on the left and select
Telnyx Environment
You should see list of variables with the ability to edit existing variables and add new variables. Please do the following steps here:
- For the
customerApiKey
variable, in the Initial Value and Current Value columns, enter your API key that you created earlier in Step 1. - Click Save at the top right to save the value to the environment.
- For the
-
Click the box in the top right corner that has list of environments and select
Telnyx Environment
from the list. Initially it shows up asNo Environment
.Doing this will set the workspace to use
Telnyx Environment
moving forward.
You are all set with the Telnyx environment for Postman.
Import collections
Postman Collections are a group of saved requests.
We made it easy to import postman collections by adding a Run in Postman Button
in all the API reference pages that allows you to fork the collections, test API requests and see the results immediately without writing any code.
For example, Use the Run in Postman button below to import the Phone Numbers API collection:
Then, it should show a dialog box with some information on benefits of forking and links to view/import the collection instead of forking. Click Fork Collection
After clicking Fork Collection, you will see a small form with some details to fill before you fork:
- Fork label: Make sure you provide a relevant label for you to easily identify it.
Eg:
telnyx's fork
- Workspace: Choose which workspace you would like this collection to be part of.
- Watch original collection: Checking this box will notify you when updates are made to the collection so you can pull the latest changes made to the collection.
Congratulations on forking the collection. You should now be able to see the collection under your selected workspace.
Send request
As you've added your API KEY
to the environment and imported a collection from the previous steps, you're now ready to send a request.
PRO TIPMake sure you have selected Telnyx Environment at the top right from the list of environments
Since we’ve imported the Phone Numbers API collection in previous steps, let’s send a request to list the phone numbers in your account:
-
Once you are in your desired workspace where you imported this collection, Select the Collections tab in Postman on the left and expand the Phone Numbers collection.
-
Expand the Number Configurations folder and select List phone numbers. This loads the List phone numbers request into Postman, ready to send.
-
Click Send. The result pane automatically displays the results of your request.
-
In case you would like to use any filters, just select the checkbox for any filter you would like from the Params tab, set the value and click Send.
If you receive an error, it's likely that one of the values in the environment isn't set correctly. Check the values and try again.
- You can play with the API and if you want to save the request with the modified parameters or any other data, just click save and it should save your changes in the collection. This change would be local to you and would not effect the original collection you forked from.
- If you would like to get the latest changes made to the original collection into your local collection, click on the Phone Numbers collection folder and then click on the three dots beside Save button which gives you a list of options. Select
Pull Changes
and it will fetch you the latest updates in that collection.
Wohoo!! You have completed your first request with Telnyx API and now you're ready to explore what all the Telnyx API has to offer.
You can find a list of all collections below.
Telnyx Postman Collections
For convenience, customers can also click one of the Run in Postman
buttons below which will automatically fork an API collection into your workspace to make API testing easier.
PRO TIPCollections below do not include the environment. Please make sure you configured your environment using the instructions here before you fork the collections using below links.
Import Telnyx API
Import any/all Telnyx API collection(s) for Postman from the following list.
Node-RED
@telnyx/node-red-telnyx
Name | Node-RED |
Author | OpenJS Foundation & Contributors |
URL | https://nodered.org |
Node-RED is an open-source flow-based programming tool that provides a visual development environment for building applications by wiring together nodes. It was originally developed by IBM Emerging Technology Services and is now part of the JS Foundation.
Node-RED allows users to create applications and services by connecting pre-built nodes together in a flowchart-like manner. Each node represents a specific task or function, such as reading data from a sensor, processing data, making decisions, or interacting with external systems. Users can drag and drop nodes onto a canvas, connect them together, and configure their properties.
The flows created in Node-RED are executed by a runtime engine, which runs on a server or device where Node-RED is installed. The runtime executes the flow in a sequential manner, passing data between nodes as it progresses through the flow. The flows can be easily modified and deployed, making it a flexible tool for building and prototyping Internet of Things (IoT) applications, automation workflows, and data integration processes.
Start the journey
node-red-telnyx is the Telnyx powerful integration that allows you to combine the capabilities of Node-RED, a flow-based programming tool, with Telnyx, a cloud-based communications platform. With node-red-telnyx, you can create complex workflows and automate various telecommunications tasks.
node-red-telnyx can be integrated with other services and platforms through Node-RED's extensive library of nodes. You can connect Telnyx's communications capabilities with databases, APIs, messaging platforms, and IoT devices to build end-to-end automation solutions.
e.g., you can use node-red-telnyx to send SMS messages programmatically. This can be useful for notifications, alerts, or two-factor authentication (2FA) purposes. You can configure the flow to trigger SMS messages based on specific events or conditions.
To get started, sign up for a Portal account, then follow the steps in our quickstart guide to buy an SMS-enabled number.
Usage
The package needs to be configured with your MCP account's API key and some other details you can find in the Telnyx Mission Control Portal.
Both ways of sending SMS are supported.
- Alphanumeric: Alphanumeric Sender ID allows you to set your company name or brand as the Sender ID when sending one-way SMS messages to international destinations. Find more information here
- Two-way: You’ll need an SMS-capable phone number purchased from, or ported into, the Telnyx platform. If purchasing a new number, select the SMS number feature when searching. In general, numbers that are ported in will be messaging-capable. More about this here
Resources
Example flows using node-red-telnyx
Getting Started Video (Coming soon)
Support
Our team provides open source support in a best effort fashion, ensuring that we offer assistance and guidance to the community based on our expertise and resources. For support, we encourage users to reach out to us through the GitHub issue reporting platform of the node-red-telnyx repository, where they can report any issues or seek help with our open source projects.
Now you know how to harness the power of Telnyx APIs for your development projects. Sign up now and dive into our comprehensive documentation to start building seamless communication experiences with ease.
You can also find example projects to get you started on GitHub:
- demo-node-telnyx
- demo-python-telnyx
- demo-ruby-telnyx
- demo-dotnet-telnyx
- demo-php-telnyx
- demo-java-telnyx
Your next innovation starts with Telnyx.